
How To... Responsive Top Navigation
Learn how to create a responsive top navigation menu with CSS and JavaScript.
Top Navigation
Resize the browser window to see how the responsive navigation menu works. To resize the browser, click on to the menu icon in the top right corner of the Firefox browser; choose "Developer" and then choose "Responsive Design View" or simply use the short cut keys "Ctrl+Shift+M".
Create A Responsive Top Navigation Menu
Step 1) Add HTML:
Example
<li><a href="#home">Home</a></li>
<li><a href="#news">News</a></li>
<li><a href="#contact">Contact</a></li>
<li><a href="#about">About</a></li>
<li class="icon">
<a href="javascript:void(0);" onclick="myFunction()"> ☰</a>
</li>
</ul>
The list item with class="icon" has a link that is used to open and close the topnav on small screens.
Step 2) Add CSS:
Style the list to look like a navigation bar:
Example
ul.topnav {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
background-color: #333;
}
/* Float the list items side by side */
ul.topnav li {float: left;}
/* Style the links inside the list items */
ul.topnav li a {
display: inline-block;
color: #f2f2f2;
text-align: center;
padding: 14px 16px;
text-decoration: none;
transition: 0.3s;
font-size: 17px;
}
/* Change background color of links on hover */
ul.topnav li a:hover {background-color: #111;}
/* Hide the list item that contains the link that should open and close the topnav on small screens */
ul.topnav li.icon {display: none;}
Add media queries:
Example
@media screen and (max-width:680px) {
ul.topnav li:not(:first-child) {display: none;}
ul.topnav li.icon {
float: right;
display: inline-block;
}
}
/* The "responsive" class is added to the topnav with JavaScript when the user clicks on the icon. This class makes the topnav look good on small screens */
@media screen and (max-width:680px) {
ul.topnav.responsive {position: relative;}
ul.topnav.responsive li.icon {
position: absolute;
right: 0;
top: 0;
}
ul.topnav.responsive li {
float: none;
display: inline;
}
ul.topnav.responsive li a {
display: block;
text-align: left;
}
}
Step 3) Add JavaScript:
Example
function myFunction() {
document.getElementsByClassName("topnav")[0].classList.toggle ("responsive");
}
Tip: Go to our CSS Navbar Tutorial to learn more about navigation bars.
How To...Create a Side Navigation Menu
Learn how to create a closable side navigation menu.
Create an Animated Side Navigation
Step 1) Add HTML:
Example
<a href="javascript:void(0)" class="closebtn" onclick="closeNav()" >×</a>
<a href="#">About</a>
<a href="#">Services</a>
<a href="#">Clients</a>
<a href="#">Contact</a>
</div>
<!-- Use any element to open the sidenav -->
<span onclick="openNav()">open</span>
<!-- Add all page content inside this div if you want the side nav to push page content to the right (not used if you only want the sidenav to sit on top of the page -->
<div id="mainContent">
...
</div>
Step 2) Add CSS:
Example
.sidenav {
height: 100%; /* 100% Full-height */
width: 0; /* 0 width - change this with JavaScript */
position: fixed; /* Stay in place */
z-index: 1; /* Stay on top */
top: 0;
left: 0;
background-color: #111; /* Black*/
overflow-x: hidden; /* Disable horizontal scroll */
padding-top: 60px; /* Place content 60px from the top */
transition: 0.5s; /* 0.5 second transition effect to slide in the sidenav */
}
/* The navigation menu links */
.sidenav a {
padding: 8px 8px 8px 32px;
text-decoration: none;
font-size: 25px;
color: #818181;
display: block;
transition: 0.3s
}
/* When you mouse over the navigation links, change their color */
.sidenav a:hover, .offcanvas a:focus{
color: #f1f1f1;
}
/* Position and style the close button (top right corner) */
.closebtn {
position: absolute;
top: 0;
right: 25px;
font-size: 36px !important;
margin-left: 50px;
}
/* Style page content - use this if you want to push the page content to the right when you open the side navigation */
#main {
transition: margin-left .5s;
padding: 20px;
}
/* On smaller screens, where height is less than 450px, change the style of the sidenav (less padding and a smaller font size) */
@media screen and (max-height: 450px) {
.sidenav {padding-top: 15px;}
.sidenav a {font-size: 18px;}
}
Step 3) Add JavaScript:
The example below slides in the side navigation, and makes it 250px wide:
Sidenav Overlay Example
function openNav() {
document.getElementById("mySidenav").style.width="250px";
}
/* Set the width of the side navigation to 0 */
function closeNav() {
document.getElementById("mySidenav").style.width = "0";
}
The example below slides in the side navigation, and pushes the page content to the right (the value used to set the width of the sidenav is also used to set the left margin of the "page content"):
Sidenav Push Content
function openNav() {
document.getElementById("mySidenav").style.width="250px";
document.getElementById("main").style.marginLeft="250px";
}
/* Set the width of the side navigation to 0 and the left margin of the page content to 0 */
function closeNav() {
document.getElementById("mySidenav").style.width="0";
document.getElementById("main").style.marginLeft="0";
}
The example below also slides in the side navigation, and pushes the page content to the right, only this time, we add a black background color with a 40% opacity to the body element, to "highlight" the side navigation:
Sidenav Push Content w/ opacity
function openNav() {
document.getElementById("mySidenav").style.width="250px";
document.getElementById("main").style.marginLeft="250px";
document.body.style.backgroundColor="rgba(0,0,0,0.4)";
}
/* Set the width of the side navigation to 0 and the left margin of the page content to 0, and the background color of body to white */
function closeNav() {
document.getElementById("mySidenav").style.width = "0";
document.getElementById("main").style.marginLeft = "0";
document.body.style.backgroundColor = "white";
}
The example below opens and close the side navigation menu without animations:
Sidenav without Animation
function openNav() {
document.getElementById("mySidenav").style.display = "block";
}
/* Close/hide the sidenav */
function closeNav() {
document.getElementById("mySidenav").style.display = "none";
}
How To... Alerts
Learn how to create alert messages with CSS.
Alerts
Alert messages can be used to notify the user about something special: danger, success, information or warning.
Create An Alert Message
Step 1) Add HTML:
Example
<span class="closebtn" onclick="this.parentElement.style.display= 'none';">×</span>
This is an alert box.
</div>
If you want the ability to close the alert message, add a <span>
element with an onclick
attribute that says "when you click on me,
hide my parent element" - which is the container <div>
(class="alert").
Tip: Use the HTML entity "×
" to
create the letter "x".
Step 2) Add CSS:
Style the alert box and the close button:
Example
.alert {
padding: 20px;
background-color: #f44336; /* Red */
color: white;
margin-bottom: 15px;
}
/* The close button */
.closebtn {
margin-left: 15px;
color: white;
font-weight: bold;
float: right;
font-size: 22px;
line-height: 20px;
cursor: pointer;
}
.closebtn:hover {
color: black;
}
Many Alerts
If you have many alert messages on a page, you can add the following script to close different alerts without using the onclick attribute on each <span> element.
And, if you want the alerts to slowly fade out when you click on them, add
opacity
and transition
to the alert
class:
Example
.alert {
opacity: 1;
transition: opacity 0.6s; // 600ms to fade out
}
</style>
<script>
// Get all elements with class="closebtn"
var close = document.getElementsByClassName("closebtn");
var i;
// Loop through all close buttons
for (i = 0; i < close.length; i++) {
// When someone clicks on a close button
close[i].onclick = function(){
// Get the parent of <span class= "closebtn"> (<div class="alert">)
var div = this.parentElement;
// Set the opacity of div to 0 (transparent)
div.style.opacity = "0";
// Hide the div after 600ms (the same amount of milliseconds it takes to fade out)
setTimeout(function(){ div.style.display = "none"; }, 600);
}
}
</script>
How To... Modal Images
Learn how to create responsive Modal Images with CSS and JavaScript.
Modal Image
A modal is a dialog box/popup window that is displayed on top of the current page.
This example use most of the code from the previous example, Modal Boxes, only in this example, we use images.
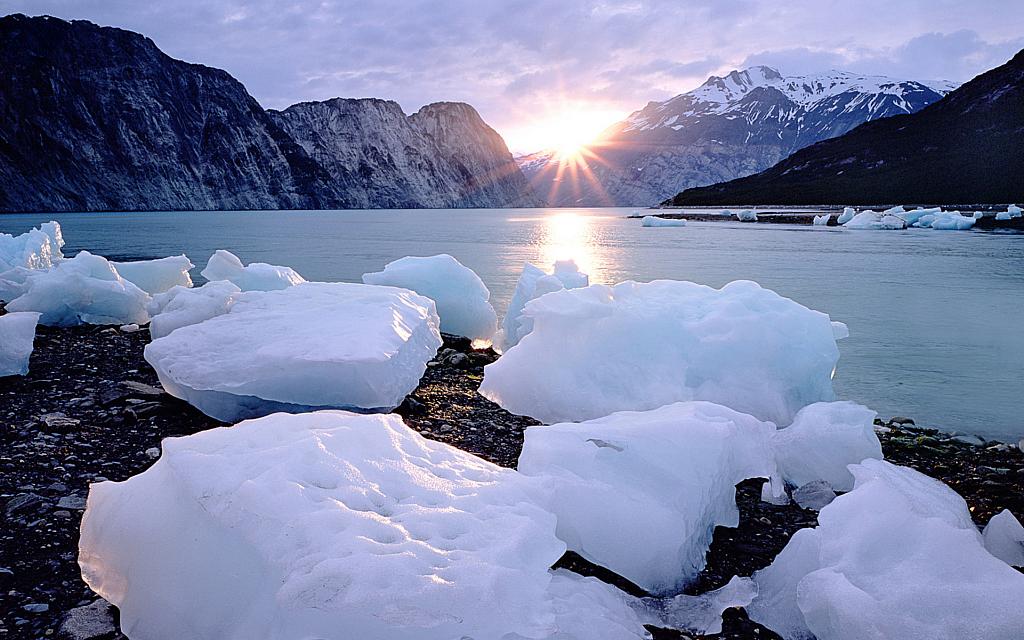
Step 1) Add HTML:
Example
<img id="myImg" src="img_fjords2.jpg" alt="Trolltunga, Norway" width="300" height="200">
<!--The Modal-->
<div id="myModal" class="modal">
<!-- The Close Button -->
<span class="close" onclick="document.getElementById('myModal') .style.display='none'">×</span>
<!-- Modal Content (The Image) -->
<img class="modal-content" id="img01">
<!-- Modal Caption (Image Text) -->
<div id="caption"></div>
</div>
Example Explained
The Trigger/Open Part
Use any element to open the actual modal, e.g. a <button> or an <a> element and specify a unique ID.
The Modal Part
The <div> with class="modal"
is a container element for
the modal and the div with class="modal-content"
is where you put
your modal content (headings, paragraphs, images, etc).
The <span> element with class="close"
should be used to
close the modal.
Step 2) Add CSS:
Example
#myImg {
border-radius: 5px;
cursor: pointer;
transition: 0.3s;
}
#myImg:hover {opacity: 0.7;}
/* The Modal (background) */
.modal {
display: none; /* Hidden by default */
position: fixed; /* Stay in place */
z-index: 1; /* Sit on top */
padding-top: 100px; /* Location of the box */
left: 0;
top: 0;
width: 100%; /* Full width */
height: 100%; /* Full height */
overflow: auto; /* Enable scroll if needed */
background-color: rgb(0,0,0); /* Fallback color */
background-color: rgba(0,0,0,0.9); /* Black w/ opacity */
}
/* Modal Content (Image) */
.modal-content {
margin: auto;
display: block;
width: 80%;
max-width: 700px;
}
/* Caption of Modal Image (Image Text) - Same Width as the Image */
#caption {
margin: auto;
display: block;
width: 80%;
max-width: 700px;
text-align: center;
color: #ccc;
padding: 10px 0;
height: 150px;
}
/* Add Animation - Zoom in the Modal */
.modal-content, #caption {
-webkit-animation-name: zoom;
-webkit-animation-duration: 0.6s;
animation-name: zoom;
animation-duration: 0.6s;
}
@-webkit-keyframes zoom {
from {transform:scale(0)}
to {transform:scale(1)}
}
@keyframes zoom {
from {transform:scale(0)}
to {transform:scale(1)}
}
/* The Close Button */
.close {
position: absolute;
top:15px;
right: 35px;
color: #f1f1f1;
font-size: 40px;
font-weight: bold;
transition: 0.3s;
}
.close:hover,
.close:focus {
color: #bbb;
text-decoration: none;
cursor: pointer;
}
/* 100% Image Width on Smaller Screens */
@media only screen and (max-width: 700px){
& nbsp; .modal-content {
width: 100%;
}
}
Example Explained
The .modal
class
The .modal
class represents the window BEHIND (background)the
actual modal box. The height and width is set to 100%, which should create the
illusion of a background window.
Add a black background color with opacity.
Set position to fixed; meaning it will move up and down the page when the user scrolls.
It is hidden by default, and should be shown with a click of a button (this will get covered more later).
The .modal-content
class
This is the actual modal box that gets focus. Do whatever you want with it.
You have a good start with a border, some padding, and a background color. The
margin: 15% auto
is used to push the modal box down from the top
(15%) and centering it (auto).
Also, the width is set to 400px - this could be more or less, depending on screen size. This will also be covered more later.
The .close
class
The close button is styled with a large font-size, a specific color and floats to the right. Styles are available that will change the color of the close button when the user moves the mouse over it.
Step 3) Add JavaScript:
Example
var modal = document.getElementById('myModal');
// Get the image and insert it inside the modal - use its "alt" text as a caption
var img = document.getElementById('myImg');
var modalImg = document.getElementById("img01");
var captionText = document.getElementById("caption");
img.onclick = function(){
modal.style.display = "block";
modalImg.src = this.src;
modalImg.alt = this.alt;
captionText.innerHTML = this.alt;
}
// Get the <span> element that closes the modal
var span = document.getElementsByClassName("close")[0];
// When the user clicks on <span> (x), close the modal
span.onclick = function() {
modal.style.display = "none";
}
How To... Animated Search Form
Learn how to create an animated search form with CSS.
How To Create an Animated Search Form
Click on the input field:
Step 1) Add HTML:
Example
Step 2) Add CSS:
Example
width: 130px;
-webkit-transition: width 0.4s ease-in-out;
transition: width 0.4s ease-in-out;
}
/* When the input field gets focus, change its width to 100% */
input[type=text]:focus {
width: 100%;
}